Servlet Introduction
Servlet (Example-1)
Life Cycle Events
Con.Ini.Par.(Example-2)
Lif.Cyc.Evt.(Example-3)
Err. & Exce.(Example-4)
Filters (Example-5)
Servlet Hints
Bookmark This Site
|
Introduction to Java Servlet (Example Program)
Introduction
|
Java Servlet is the one of the most important Java technologies. It is the simplest model to build a complete Java J2EE Web Application. Furthermore, even for complex J2EE Web Application that uses Struts, Spring, EJB and etc, they are still using Servlet for certain purposes such as Servlet Filter, Listener and etc. Thus, it is just a good idea for you to have well-built understanding of Java Servlet. Prior reading this tutorial, it would be excellent if you have mastered the basic Java programming languages.
Servelet Example is give below with complete steps with screen-shots
CLICK HERE to download this complete example (zip file)
|
|
Create a User Interface Using HTML
|
-
<html>
-
<head>
-
<title>
-
Find Employee Information
-
</title>
-
</head>
-
<body>
-
<form method="get" action="http://localhost:8080/emp_details">
-
<h2 align=center>Find Employee Information<center></h2>
-
<table>
-
<tr>
-
<th>Enter Employee Id>/th>
-
<td><input type=text name="id"></td>
-
</tr>
-
</table>
-
<input type=submit value=Submit>
-
</form>
-
</body>
-
</html>
Download: Employee.html
|
Servlet Coding
|
import java.io.*;
import java.sql.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class EmployeeDetails extends HttpServlet{
static int i;
Connection con;
PrintWriter out;
ResultSet rs;
public void init(){
i=0;
con=null;
out=null;
rs=null;
}
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException{
i++;
out=response.getWriter();
out.println("<b>You are user number " + i + "to visit this site</b><br><br>");
try{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
con=DriverManager.getConnection("jdbc:odbc:EmployeesDB","sa","franklin");
PreparedStatement pstmt=null;
String query=null;
query="select emp_fname,address,age,desig from Employee_Master where id=?";
pstmt=con.prepareStatement(query);
pstmt.setInt(1,Integer.parseInt(request.getParameter("id")));
rs=pstmt.executeQuery();
out.println("<b><center>Employee Details</center></b><br><br>");
ResultSetMetaData rsmd=rs.getMetaData();
int colcount=rsmd.getColumnCount();
out.println("<table align=center border=1 cellpadding=2>");
out.println("<tr>");
for(int i=1; i<=colcount; i++){
out.println("<th>" + rsmd.getColumnLabel(i) + "</th>");
}
out.println("<tr>");
while(rs.next()){
out.println("<tr>");
out.println("<td>" + rs.getString("emp_fname") + "</td>");
out.println("<td>" + rs.getString("address") + "</td>");
out.println("<td>" + rs.getString("age") + "</td>");
out.println("<td>" + rs.getString("desig") + "</td>");
out.println("</tr>");
}
out.println("</table>");
out.println("</body>");
}
catch(Exception e){
out.println(e.toString());
}
}
public void destroy(){
try{
i=0;
con.close();
out.close();
rs.close();
}
catch(SQLException se){
out.println(se.toString());
}
}
}
Download: EmployeeDetails.java
|
Package the Servlet into a J2EE Application
|
-
Write a html file and name it as “Employee.html”
-
Write a java program and name it as “EmployeeDetails.java”
-
Set the path in the “command prompt”
-
set path=.;C:\progra~1\java\j2sdk1.5.0\bin;C:\Sun\AppServer\bin;
-
Set classpath=.;C:\progra~1\java\j2sdk1.5.0\lib;C:\Sun\AppServer\lib\j2ee.jar;
(OR) Set the path in the system itself. CLICK HERE for details
-
Now compile the “EmployeeDetails.java”. CLICK HERE to see how to compile
-
After the java programs are compiled successfully, you can close the command prompt.
- Check whether the MSSQL Server is running. If the MSSQL Server is not running then you need to start the MSSQL Server first (CLICK HERE for details)
-
Now open the SQL Query Analyzer (CLICK HERE for details)
Now type the following queries -
Create table Employee_Master(emp_fname varchar(20), address varchar(20), age int, desig varchar(20), id varchar(20));
-
Insert into Employee_Master values(‘Franklin’, ‘Pooteti’, 25, ‘MCA’, ‘1’);
-
Close the SQL Query Analyzer
-
Goto Control Panel (Start -> Settings ->Control Pannel)
-
Click Administrative Tools (in XP, click Performance and Maintenance) and then click Data Sources (ODBC)
-
Now
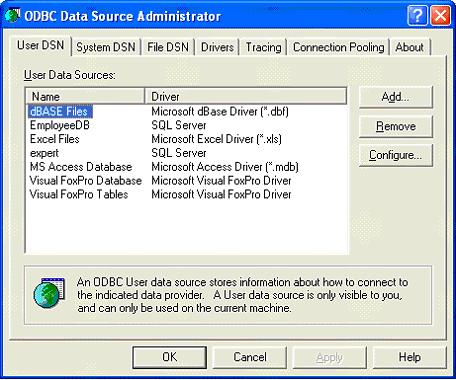 Click the "Add" button
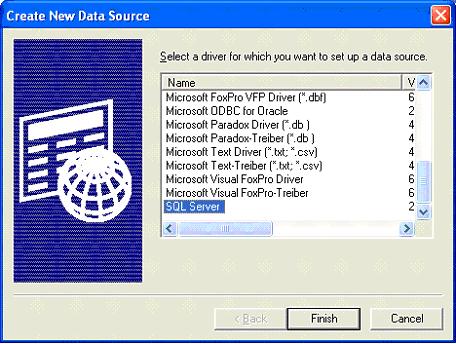 Select “SQL Server” and click Finish
| (OR) | |
Write “EmployeesDB” in the Name textbox. Then in the Server text box type the server name (CLICK HERE for server-name) (or) type . as shown in the image above
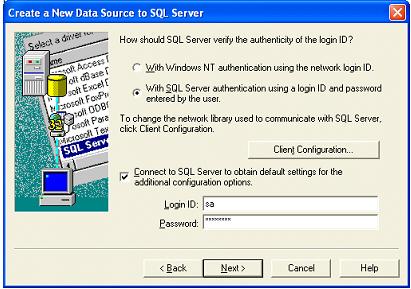 Now Select the option “With SQL Server authentication using a login ID and password entered by the user”. Then select “Connect to SQL Server to obtain default settings for the additional configuration options”
Then in the Login ID text box type sa and in the Password text box type the password (CLICK HERE for password). I am using franklin in the Password text box. And then press the Next button
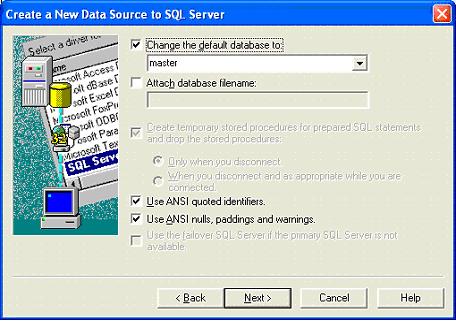 Select the Change the default database to and give your database name (Optional)
Next click the Next button
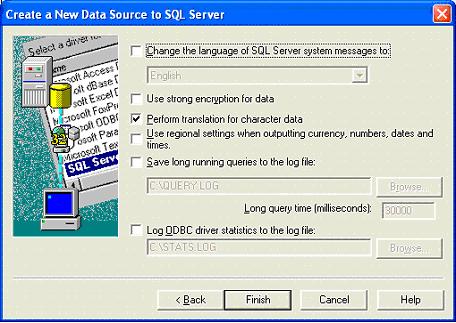 Click the Finish button
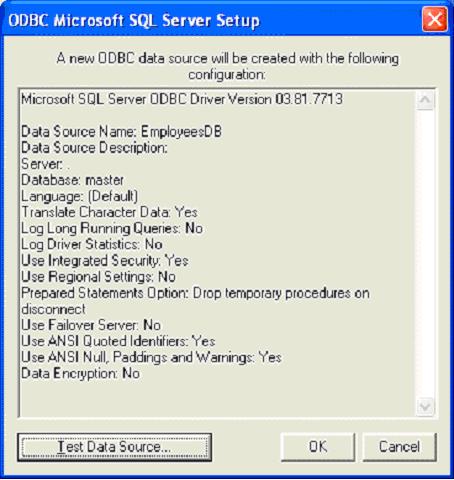 Click Text Data Source button
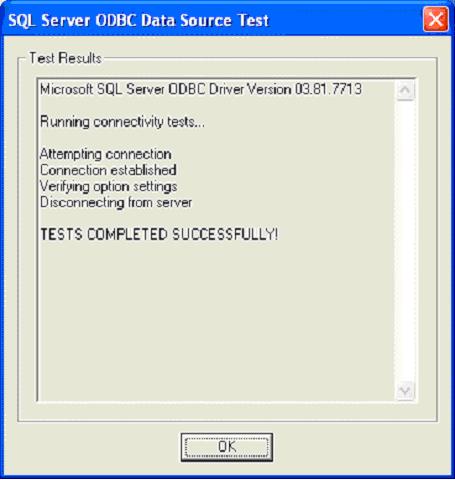 Press OK button
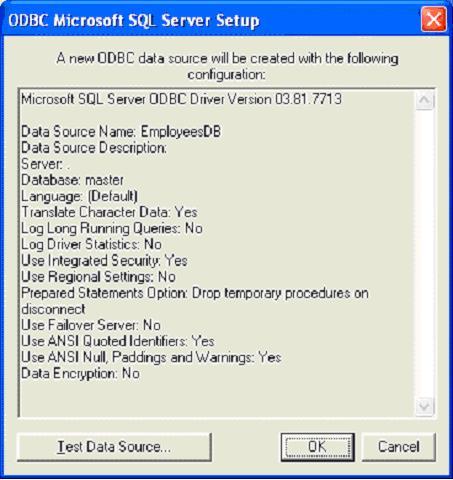 Press OK button
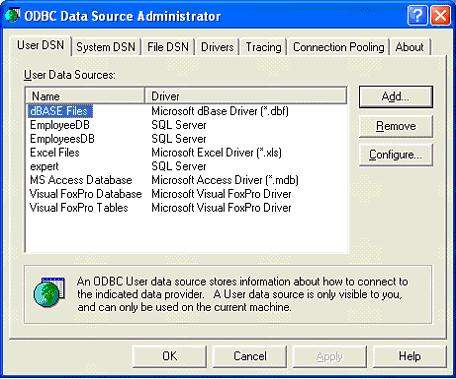 Press OK button
-
Now goto Start -> Programs -> Sun Microsystems ->Application Server PE -> Start Default Server (wait till it start and then press any key). CLICK HERE to see how to Start the Server
|
|
Click Next To Continue ...
|
|
|